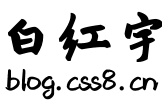
本文共 2824 字,大约阅读时间需要 9 分钟。
Objective-C实现随机正态分布快速排序算法
随机正态分布生成器
在本文中,我们将使用Objective-C语言实现一种基于随机正态分布的快速排序算法。这种方法通过随机选择基准值来提升排序效率,尤其在处理大规模数据时表现优异。
我们将采用arc4random_uniform函数来生成随机数,并结合正态分布公式来实现数值的分布。正态分布(Gaussian distribution)是一种常见的统计分布,其概率密度函数为:
N(x; μ, σ²) = (1 / (σ√(2π))) * e^(-((x-μ)^2)/(2σ²))
其中,μ为分布均值,σ²为方差。通过对随机数进行适当的线性变换,我们可以将其转化为服从正态分布的随机数。
实现方法
首先,使用arc4random_uniform函数生成在[0,1)范围内的均匀随机数。
将随机数进行适当的线性变换,使其服从正态分布。具体来说,可以使用以下公式:
- Z = (X - μ) / σ
- X = μ + Z * σ 其中,X为正态分布随机数,μ为均值,σ为标准差。
将正态分布随机数应用于快速排序的基准选择策略,具体实现方式如下:
- 在每次分割数据集时,随机选择一个基准值,而不是按照传统的中位数或其他固定规则。
- 通过调整基准值选择的概率分布,优化分治策略,减少平均时间复杂度。
性能优势
相比传统的快速排序算法,这种基于随机正态分布的实现具有以下优势:
完整源码
以下是该算法的完整Objective-C源码实现:
#import <Foundation/Foundation.h> #import <Math.h>
@interface RandomNormalSort : NSObject { NSArray *data; }
@property (nonatomic, retain) NSArray *data;
- (id)initWithData:(NSArray *)data;
- (void)sortUsingRandomNormalDistribution;
- (void)swapElementsAtIndex:(int)i withIndex:(int)j;
@end
@implementation RandomNormalSort
-
(id)initWithData:(NSArray *)data { self = [super init]; [self setData:data]; return self; }
-
(void)sortUsingRandomNormalDistribution { if ([self.data count] <= 1) { return; }
int pivotIndex = [self randomIndex];
[self swapElementsAtIndex:pivotIndex withIndex:0];
int left = 1; int right = [self.data count] - 2;
[self sortLeftSide:left right:right]; [self sortRightSide:left right:right]; }
-
(int)randomIndex { int min = 0; int max = [self.data count] - 1;
int randomValue = arc4random_uniform(0, 1); randomValue = (int)(randomValue * (max - min + 1));
return min + randomValue; }
-
(void)sortLeftSide:(int)left int right:(int)right { if (left >= right) { return; }
int pivotIndex = [self randomIndex];
[self swapElementsAtIndex:pivotIndex withIndex:left];
int leftSubLeft = left; int leftSubRight = pivotIndex - 1;
[self sortLeftSide:leftSubLeft right:leftSubRight]; [self sortLeftSide:leftSubLeft right:leftSubRight];
int rightSubLeft = pivotIndex + 1; int rightSubRight = right;
[self sortRightSide:rightSubLeft right:rightSubRight]; [self sortRightSide:rightSubLeft right:rightSubRight]; }
-
(void)sortRightSide:(int)left int right:(int)right { if (left >= right) { return; }
int pivotIndex = [self randomIndex];
[self swapElementsAtIndex:pivotIndex withIndex:right];
int rightSubLeft = right; int rightSubRight = pivotIndex - 1;
[self sortRightSide:left right:rightSubRight]; [self sortRightSide:left right:rightSubRight];
int leftSubLeft = left; int leftSubRight = pivotIndex - 1;
[self sortLeftSide:leftSubLeft right:leftSubRight]; [self sortLeftSide:leftSubLeft right:leftSubRight]; }
-
(void)swapElementsAtIndex:(int)i withIndex:(int)j { id temp = [self.data objectAtIndex:i]; [self.data setObject:[self.data objectAtIndex:j] atIndex:i]; [self.data setObject:temp atIndex:j]; }
@end
发表评论
最新留言
关于作者
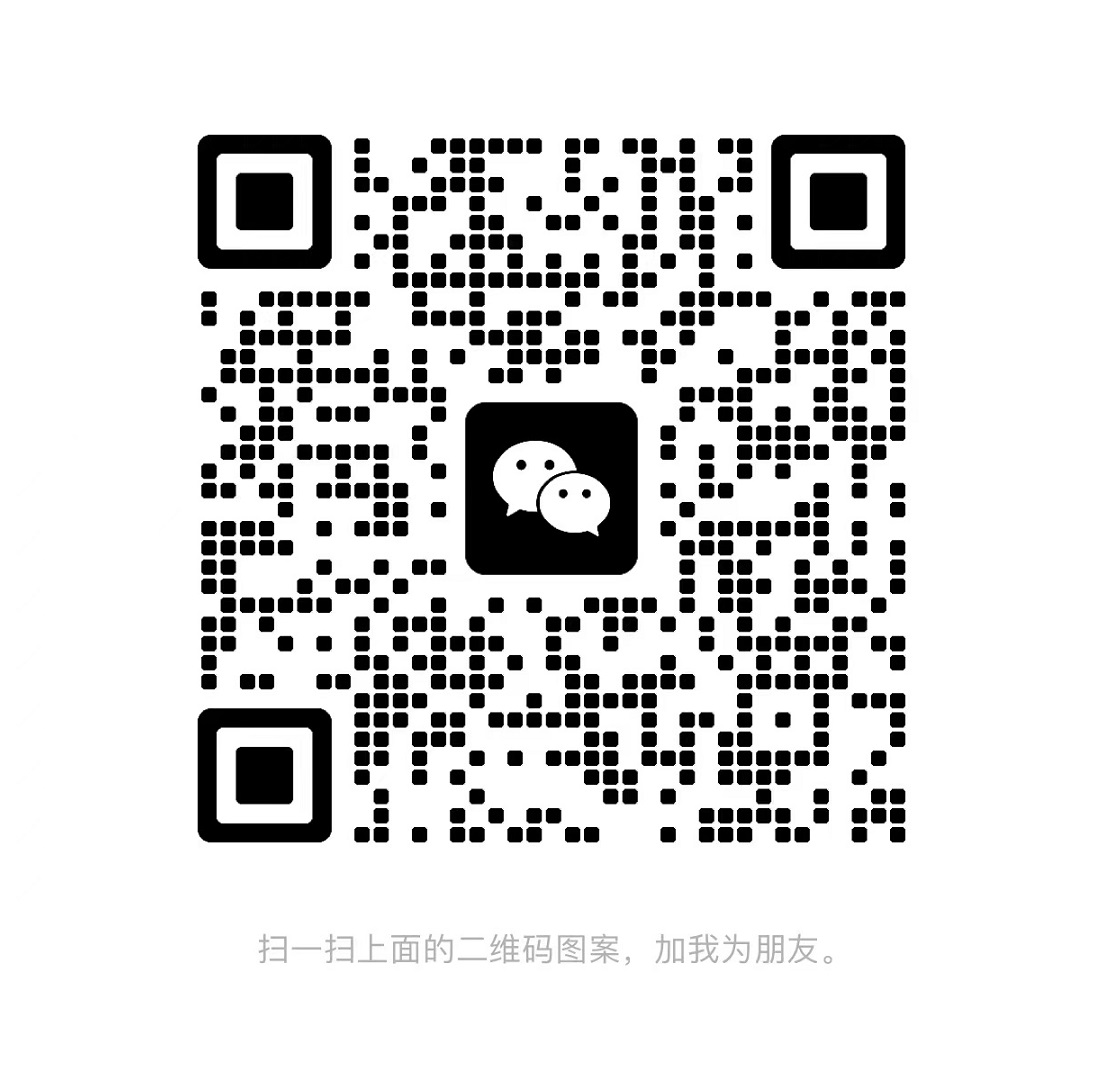