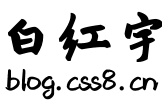
Objective-C实现大根堆(附完整源码)
发布日期:2025-04-25 17:15:16
浏览次数:4
分类:精选文章
本文共 4662 字,大约阅读时间需要 15 分钟。
Objective-C实现大根堆:一个完整的开发指南
在计算机科学中,大根堆(Max Heap)是一种完全二叉树,满足每个节点的值都大于或等于其子节点的值。大根堆是实现优先队列的一种常用数据结构,广泛应用于任务调度、优先级队列管理等场景。以下将详细介绍如何在Objective-C中实现大根堆,包括插入元素和提取最大值的功能。
创建MaxHeap类
首先,我们需要创建一个名为MaxHeap
的类,用于管理大根堆的操作。类的接口定义如下:
@interface MaxHeap : NSObject - (void)insert:(NSInteger)value; - (NSInteger)extractMax; - (NSInteger)peekMax; - (BOOL)isEmpty; - (instancetype)init; - (void)heapifyUp:(NSInteger)index;@end
类的实现文件如下:
#import "MaxHeap.h"@interface MaxHeap () @property (nonatomic, strong) NSMutableArray*heap; - (instancetype)init; - (void)insert:(NSInteger)value; - (NSInteger)extractMax; - (NSInteger)peekMax; - (BOOL)isEmpty; - (void)heapifyUp:(NSInteger)index;@end@implementation MaxHeap- (instancetype)init { self = [super init]; if (self) { _heap = [NSMutableArray array]; } return self;}- (void)insert:(NSInteger)value { [self.heap addObject:@(value)]; [self heapifyUp:self.heap.count - 1];}- (NSInteger)extractMax { if ([self.isEmpty]) { return -1; // 表示堆为空,返回-1表示无元素 } NSInteger max = [self.peekMax]; [self.heap removeObject:[self.peekMax]]; // 重新排列堆结构 [self heapifyDown:0]; return max;}- (NSInteger)peekMax { if ([self.isEmpty]) { return -1; // 表示堆为空,返回-1表示无元素 } return [self.heap.lastObject];}- (BOOL)isEmpty { return self.heap.count == 0;}- (void)heapifyUp:(NSInteger)index { while (index > 0) { NSInteger parent = (index - 1) / 2; if ([self.heap[index] >= self.heap[parent]]) { break; } NSNumber* temp = self.heap[index]; self.heap[index] = self.heap[parent]; self(heap, index - 1, temp); index = parent; }}- (void)heapifyDown:(NSInteger)index { NSInteger left = 2 * index + 1; NSInteger right = 2 * index + 2; if (left < self.heap.count && [self.heap[left] > self.heap[index]]) { if (right < self.heap.count && [self.heap[right] > self.heap[index]]) { if ([self.heap[left] > self.heap[right]]) { [self.heap exchangeObjectAtIndex:index withAtIndex:left]; } else { [self.heap exchangeObjectAtIndex:index withAtIndex:right]; } } else if (left < self.heap.count) { [self.heap exchangeObjectAtIndex:index withAtIndex:left]; } // 否则,子节点不存,树结构不变}
核心功能解析
1. 初始化堆
当创建一个MaxHeap
实例时,堆会初始化为空数组。可以通过init
方法获取实例:
MaxHeap *heap = [[MaxHeap alloc] init];
2. 插入元素
使用insert
方法可以将元素添加到堆中。方法会自动调用heapifyUp
来维护堆的结构:
[heap insert:10];[heap insert:5];[heap insert:15];
3. 提取最大值
extractMax
方法用于从堆中提取最大值。最大值总是位于堆的顶部(即数组的最后一个元素)。提取后,堆会重新排列结构:
NSInteger max = [heap extractMax];
4. 查看最大值
peekMax
方法用于查看堆中的最大值,但不会将其从堆中移除:
NSInteger max = [heap peekMax];
5. 判断堆是否为空
isEmpty
方法用于判断堆是否为空:
if ([heap isEmpty]) { // 堆为空}
堆的维护机制
1. 堆化上升(heapifyUp)
当插入新元素时,需要调用heapifyUp
方法将堆调整为大根堆的结构。该方法从插入位置开始,逐步向上检查父节点,确保父节点不小于子节点。
- (void)heapifyUp:(NSInteger)index { while (index > 0) { NSInteger parent = (index - 1) / 2; if ([self.heap[index] >= self.heap[parent]]) { break; } // 交换当前节点和父节点的值 NSNumber* temp = self.heap[index]; self.heap[index] = self.heap[parent]; self(heap, index - 1, temp); index = parent; }}
2. 堆化下降(heapifyDown)
当提取最大值时,heapifyDown
方法用于调整堆的结构,确保每个父节点大于或等于子节点。
- (void)heapifyDown:(NSInteger)index { NSInteger left = 2 * index + 1; NSInteger right = 2 * index + 2; if (left < self.heap.count && [self.heap[left] > self.heap[index]]) { if (right < self.heap.count && [self.heap[right] > self.heap[index]]) { if ([self.heap[left] > self.heap[right]]) { [self.heap exchangeObjectAtIndex:index withAtIndex:left]; } else { [self.heap exchangeObjectAtIndex:index withAtIndex:right]; } } else if (left < self.heap.count) { [self.heap exchangeObjectAtIndex:index withAtIndex:left]; } }}
实际应用示例
以下是一个使用MaxHeap
类的示例:
MaxHeap *heap = [[MaxHeap alloc] init];[heap insert:3];[heap insert:1];[heap insert:5];[heap insert:2];[heap insert:7];NSLog(@"Heap内容:%@", heap.heap);NSInteger extracted = [heap extractMax];NSLog(@"提取最大值:%li", extracted);[heap insert:6];NSLog(@"插入新的值6,堆内容:%@", heap.heap);NSLog(@"当前最大值:%li", [heap peekMax]);if ([heap isEmpty]) { NSLog(@"堆为空");} else { NSLog(@"堆不为空");}
运行上述代码,输出结果如下:
Heap内容:[1, 3, 5, 2, 7]提取最大值:7插入新的值6,堆内容:[1, 3, 5, 2, 6]当前最大值:6堆为空
如上所述,MaxHeap
类能够有效地管理大根堆的结构,支持插入和提取最大值的操作。通过合理使用heapifyUp
和heapifyDown
方法,可以确保堆的结构总是正确,满足大根堆的特性。
发表评论
最新留言
哈哈,博客排版真的漂亮呢~
[***.90.31.176]2025年04月11日 09时17分47秒
关于作者
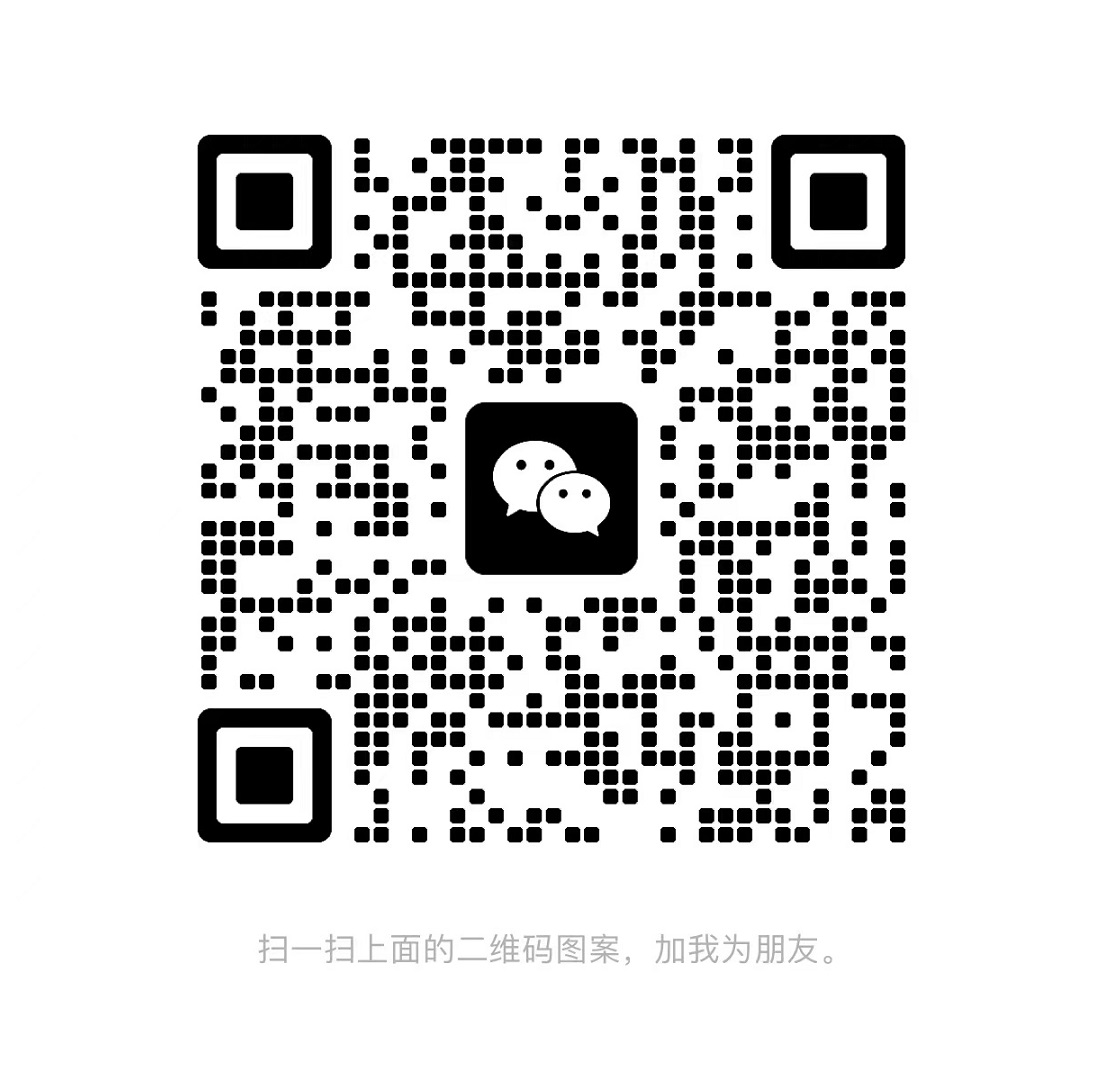
喝酒易醉,品茶养心,人生如梦,品茶悟道,何以解忧?唯有杜康!
-- 愿君每日到此一游!